Let’s start writing some programs in Go that will allow us to pass command-line arguments when running the program. It is really easy and allows us to pass custom arguments when running a Go program. We will create our custom arguments as well.
Working with command-line arguments
How to pass the arguments? Simply put those in a space-separated way alongside with program name.
./program-name -a b –c=d
1. The “os” package
The os package contains the Args array, a string array that contains all the command-line arguments passed. Let’s see our first argument, the program name which you don’t need to pass at all.
The following code will print the program name in the command line.
package main
import (
"os"
"fmt"
)
func main() {
// the first argument is always program name
programName := os.Args[0]
fmt.Println(programName)
}

2. Number of CLI arguments
We can get the number of arguments passed through the command-line easily. To do that, we use len() function.
Here is the code that prints the length of arguments passed.
package main
import (
"fmt"
"os"
)
func main() {
argLength := len(os.Args[1:])
// use fmt.Printf() to format string
fmt.Printf("Arg length is %d", argLength)
}

We are using slicing to have all arguments other than the first one.
3. Iterating over command-line arguments passed
To iterate over the arguments passed via the command line, we will loop over the os.Args array.
Below, you can see how to do that.
package main
import (
"fmt"
"os"
)
func main() {
// the first argument i.e. program name is excluded
argLength := len(os.Args[1:])
fmt.Printf("Arg length is %d\n", argLength)
for i, a := range os.Args[1:] {
fmt.Printf("Arg %d is %s\n", i+1, a)
}
}

Creating our custom arguments
Now, we will create flags to implement custom CLI arguments.
4. The “flag” package
To implement flags, we will use a package called flag. We will create a faux login mechanism as an example.
Below is the code for the faux login system.
package main
import (
"flag"
"fmt"
)
func main() {
// variables declaration
var uname string
var pass string
// flags declaration using flag package
flag.StringVar(&uname, "u", "root", "Specify username. Default is root")
flag.StringVar(&pass, "p", "password", "Specify pass. Default is password")
flag.Parse() // after declaring flags we need to call it
// check if cli params match
if uname == "root" && pass == "password" {
fmt.Printf("Logging in")
} else {
fmt.Printf("Invalid credentials!")
}
}

5. Inserting wrong values
Inserting wrong values will give you an error and provide you the correct usage of those flags. For example, if you input a number while using a string flag, an error will be thrown and the correct usage will be printed to the console.
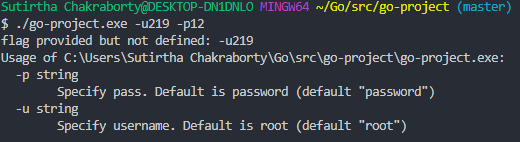
6. Creating custom usage
We can use flag.Usage to define custom usage string which will be shown after the wrong usage. So, we can customize how it is shown.
package main
import (
"flag"
"fmt"
)
func main() {
var name string
flag.StringVar(&name, "n", "admin", "Specify name. Default is admin.")
flag.Usage = func() {
fmt.Printf("Usage of our Program: \n")
fmt.Printf("./go-project -n username\n")
// flag.PrintDefaults() // prints default usage
}
flag.Parse()
}
