Logging is essential when creating large software that has a complicated flow of execution. In multiple stages of execution, it allows us to debug the program for errors. In this post, we are going to explore the Golang log package and what can we do with it.
Importing Golang log Package
To use logging in Go, we need to import the log package.
import "log"
The logging functions
The log package consists of logging functions that allow us to log data at various segments of a program. Here are some of the functions discussed in detail.
1. The Fatal function
The fatal function is just like the print function the difference is that it calls os.Exit(1)
at the end. Here are all of the Fatal functions in action.
package main
import (
"log"
)
func main() {
log.Fatal("Exception occured!") // 2020/02/15 08:52:06 Exception occured!
}
The Fatal function writes the date and time automatically before logging.

The Fatalf and Fatalln are similar with little differences. Here is an example showing these functions.
package main
import (
"log"
)
func main() {
s := "formatted"
log.Fatalf("A %s string for logging", s)
}

package main
import (
"log"
)
func main() {
log.Fatalln("A logged string with a newline")
}

The date and time come from a type called flags, which are already set up to provide the needed formatting for a logged string. The current active flag is Ldate|Ltime.
2. The Panic functions
The panic functions end the execution by throwing a panic. Panic occurs when unexpected things happen in code.
package main
import (
"log"
)
func main() {
// uncomment the lines to try different functions
// log.Panic("Error!!")
// s, v := "formatted", 42
// log.Panicf("A %s Error with a num %d!!", s, v)
log.Panicln("A panic with a newline!")
}
The output of those lines is as follows, with panic being the most straightforward and panicf and panicln supporting formatting and a newline respectively.
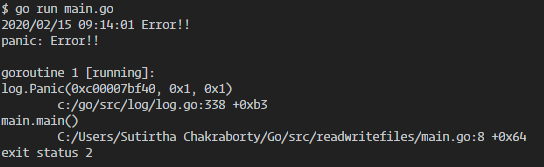
For panicf:
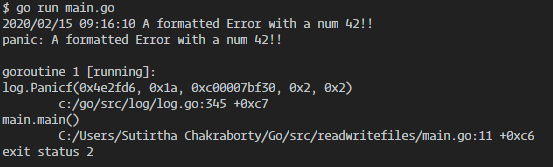
For panicln:
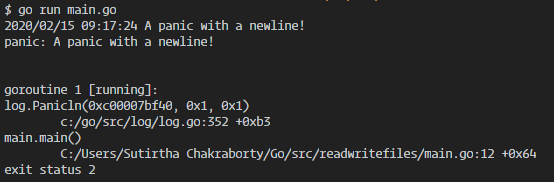
3. The print functions
The print functions are just like regular print functions i.e Print, Printf and Println and the only difference being that the flags will be set before. The LstdFlags is set i.e we get the standard flags before. We can set different flags for different outputs.
4. Set output flags
The SetFlags function sets the flags for output. The flags are to be set as an integer. Here is the chart for the flags.
log.SetFlags(0) // no flags
log.SetFlags(1) // 2020/02/15
//OR-ing flags
log.SetFlags(2 | 3) // 2020/02/15 10:56:11
The logger type
The logger is a type defined as a struct in the package. It is an object which can log into the io.Writer.
The logging package is a versatile package that can be used in many different situations and it is an important package in the Go ecosystem.