Go offers benchmarking via the testing package. In this post, we will try doing simple benchmarks to see how it works.
What is a Benchmark?
A benchmark by the dictionary definition is a standard, with which the same type of things may be compared. In programming, benchmark tests the time of execution for an operation. For example, a complex function execution time or a simple function executed a million times can be considered for benchmarking.
Required imports for Benchmarking in Go
The testing package has benchmark functions which can be used directly to produce benchmarks.
import "testing"
Benchmark rules in Go
To do benchmark the functions defined for the benchmark must be of the form as shown below.
func BenchmarkXXX(b *tesing.B){
// do benchmark...
}
When running the go test
we must provide the -bench
flag which will show the output.
go test -bench=. // the dot is the regex matching everything
Example benchmarks
Now, we will do some benchmarks. The first one is rather simple. It will simply test the Sprintf method. And output the result.
package main
import (
"fmt"
"testing"
)
func BenchmarkF(b *testing.B) {
for i := 0; i < b.N; i++ {
fmt.Sprintf("Hi")
}
}
The b.N is a variable to be adjusted by Go. Go chooses it after doing some small adjustments based on its internally defined rules.
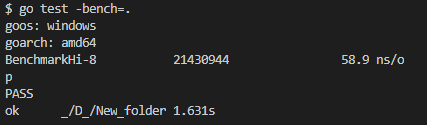
The number of times the function is executed can be seen before the average time which is 58.9ns. It is the final value of b.N.
Now, we will do a more complex example by using an array. We will try allocating an integer array containing multiples of 3. Here is the code for that benchmark.
package main
import "testing"
func f() {
var a []int
for i := 0; i < 100; i++ {
_ = append(a, i*3)
}
}
func BenchmarkF(b *testing.B) {
for i := 0; i < b.N; i++ {
f()
}
}
This benchmark produces output like this:
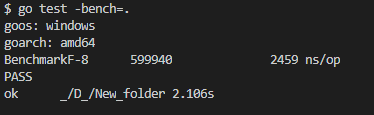
As can be seen, it is a pretty expensive function and takes much more time than before.
Importance of benchmarks
Benchmarks produce realistic expectations for the runtime of a program. It sheds light on the amount of time it can take when doing some expensive operations. It also shows how many of an operation can be done in a specific timeframe. It is really a very important metric through which many things regarding performance can be obtained that are otherwise hard to get.