Code coverage is the way of determining how much of the code is actually covered through the tests. This metric is extremely useful in determining what other tests need to be written. This post will explore code coverage in Golang.
What is code coverage?
Code coverage is the way to determine the test coverage of a package. Test coverage is a metric that provides an outline of how much of the functions are covered by tests.
The rules to follow
To do test coverage in Go we create a test file by adding a _test suffix.
filename_test.go
Then we simply use test functions in that file. To generate the coverage report, the -cover flag is added after that.
go test -cover
This cover flag tells the test tool to generate coverage reports for the package we are testing.
Coverage simple examples
Here, we are going to see some simple coverage reports generated by the coverage tool. First, we use a simple file containing a single function and test it in the test function. Since we have tested it the coverage report will say the coverage is 100%. That means all the functions are covered through tests.
The main file contains:
package main
func f() int {
return 2
}
//func g() int {
// return 4
//}
func main() {
}
The test file is as follows:
package main
import "testing"
func TestF(t *testing.T) {
v := f()
if v != 4 {
t.Error("Fails")
}
}
The file contains a simple test for the function. When we run the coverage command we get an output like this:
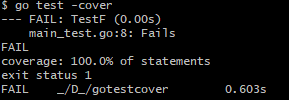
As can be seen, the test coverage is 100%. Now, let’s uncomment the other function and see the coverage report. We will see something like this:
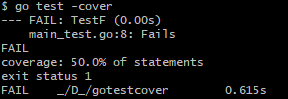
The test coverage dropped to 50%. It was expected as there are two functions but only one was covered through the test. This is what test coverage does, it calculates the percentage test done on the total number of functions and produces output accordingly.
Go Code Coverage HTML Report
We can get the coverage report in a graphical way via HTML. First, we need to set the cover profile. To do that use the command as shown below:
go test -coverprofile=coverage.out // coverage.out is the output filename
Now, we can use the following command to generate a graphical coverage report.
go tool cover -html=coverage.out
The coverage graphic will look like this:
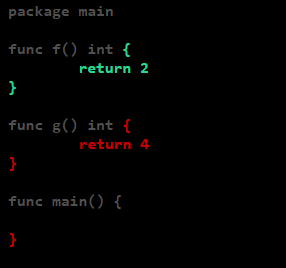
As can be seen, the graphical way of viewing the coverage is a pretty handy feature Go provides.
Code coverage importance
After the unit testing is set up code coverage is an important aspect that needs to be focused. It will make the unit tests better and will work as a complement to the tests.