Unit testing is essential when creating software. It tests each component one after another but does not test it entirely. In this post, we will use the testing package to do some unit testing in Go.
What is testing?
Testing is when we want to check the correctness of our code. It’s a really important step in software development. This post will explore the testing package for unit testing.
Testing rules
To write unit tests in Golang, we need to import the testing package. There are some rules when doing testing. The filename must end with _test
.
http_test.go // example filename for testing
The command to be used is go test
in the CLI. It will test all the files set up as test files and return the output in the terminal.
The functions in that test file must follow this signature.
func TestXXX(t *testing.T) {
// do testing
}
Testing our code
Let’s start testing some simple code. Create a file with a suffix _test. Then write the code as shown below.
package main
import "testing"
import "math"
func TestMathBasics(t *testing.T) {
v := math.Min(10, 0)
if v != 0 {
t.Error("Failed the test!")
}
}
In the code above, we are using the default math library to test the Min function. If that succeeds then we get output like this.

The output suggests that all the test has been “PASSED”.
Now, we will see what happens if our test fails. The below code provides a function that outputs incorrect and we test that function.
package main
import "testing"
//import "math"
// returns wrong min output
func WrongMin(x, y float64) float64 {
if x > y {
return x
} else {
return y
}
}
func TestMathBasics(t *testing.T) {
v := WrongMin(10, 0)
if v != 0 {
t.Error("Failed the test!")
}
}
If we run go test
we can see output like this.
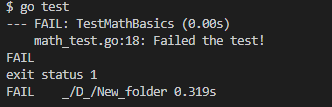
This suggests that the test FAILED due to the fact that the required conditions don’t match.
The need for testing
Testing is essential as it helps produce the correct code that otherwise will be full of errors. When we are unsure of whether the program produces the correct result the testing can help solve that problem. It is one of the most needed aspects of computer programming.
Further Reading: Makefiles in Golang