I hope everyone is finding the other Golang articles quite useful. If you need a basic fundamentals tutorial or a refresher on other functionalities in Golang, be sure to check those out. So today, we’re going to dive into the Golang OS package. I would also like to introduce you to a temporary Golang notebook on Google Colaboratory: Colab-go, where I shall be demonstrating today’s examples.
Golang OS Package – Open and Read a file
Run the first code block from the colab notebook above, and then refresh the page (don’t restart runtime !), and we’re set. Now, we know that http.Get can download us files from any URL, so let’s use that:
import (
"net/http"
"io"
"os"
)
out, _ := os.Create("novel.txt") //give any name
defer out.Close()
resp, _ := http.Get("http://www.gutenberg.org/files/18581/18581.txt")
defer resp.Body.Close()
n, _ := io.Copy(out, resp.Body)
Here, I have downloaded a free novel called “Adrift in New York” by Horatio Alger, from Project Gutenberg. You can download any file that you may see fit. The following OS functions have been used:
- os.Create() – creates an empty file in your root directory, i.e, ‘/content/’ on Colab ,or your home directory if you’re working on your PC.
- http.Get() – downloads your URL as bytes
- io.Copy() – copies the bytes from your download into the empty contents of the file created.
I’m using underscore to denote the error, which is a personal preference. Next, let’s open up our file:
import (
"os"
"log"
"fmt"
)
file, _ := os.Open("/content/novel.txt") // For read access.
if _ != nil {
log.Fatal(_)
}
The only function used here is os.Open(), which opens the file. However, it doesn’t read it yet. Also, if there’s an error with the file open, we get a log saying “No such file or directory”. Let’s now read the file, which works like a low level language in that it reads the file as byte slices. So we need to define a byte slice (say 100 bytes):
data := make([]byte, 100)
count, _ := file.Read(data)
if _ != nil {
log.Fatal(err)
}
And then we can print the first 1000 bytes of data from our file:
fmt.Printf("read %d bytes: %q\n", count, data[:count])
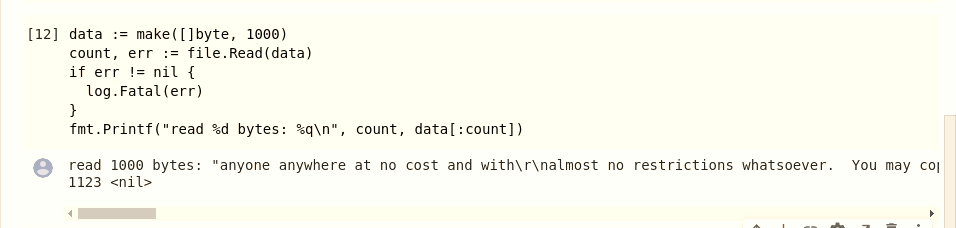
Make and Change directories using Go OS Package
Next thing we need to know is how to create and change directories, which will be required in something as basic as creating log files. There are two functions for making directories : Mkdir and MkdirAll.
The syntax for both is
func Mkdir(path_name String, perm FileMode) error
The path_name is the directory name that you’re creating.
- Mkdir creates a new directory with the specified name, otherwise throws a *PathError
- MkdirAll creates a directory named path, along with any necessary parents, and returns nil, or else returns an error.
For the second argument perm of the function, it is a number input that denotes the read/write/execute permissions of the directory. Use the Permisssions calculator for the number. Generally, 0700 is fine, which means read, write and execute permissions for the user.

To change directories, we use os.Chdir :
func Chdir(dir string) error
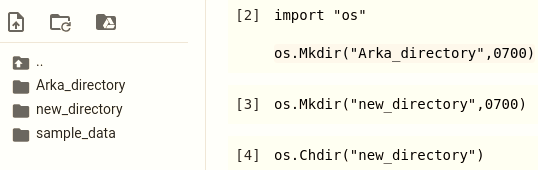
Other important OS Package functions
func Clearenv() – used to clear ALL environment variables
func Environ() []string – returns a copy of strings representing the environment, in the form “key=value”.

func Getwd() – returns a rooted path name corresponding to the current directory.
func Hostname() – returns the host name reported by the kernel.
func Remove(name) – removes the named file or (empty) directory. If there is an error, it will be of type *PathError.
func RemoveAll(name) – removes all directories and subdirectories. If the path does not exist, RemoveAll returns nil (no error). If there is an error, it will be of type *PathError.
func Rename(old_path, new_path) – renames (moves) oldpath to newpath. If newpath already exists and is not a directory, Rename replaces it.
func SameFile(file1, file2) – checks if both files are the same by comparing bytes.
That does it with 90% of the functions we’ll use day-to-day.
Leaving notes
If you want to check out the full list of obscure functions in the os package, feel free to do so https://pkg.go.dev/os.
Keep practicing. Until next time !