Hello, Gophers. How are things? First I explain about what yaml actually is, and then we’ll get into some coding in Go. So first things first.
What is YAML ?
YAML stands for YAML Ain’t Markup Language, which is a ridiculous name. But before understanding what it is, we then have to look at what markup languages are:
Markup languages make our current version of the Internet possible. Markup languages describe how text is to be displayed on a Web page or in a printed document. In a markup language, the instructions are in the form of a set of tags or special characters. For example, <Bold-On>Hello<Bold-Off> are markups, officially called tags, that will cause the word Hello to appear in bold. Markup languages do not provide interactive functionality. They serve as containers for text and graphics.
SGML (Standard Generalized Markup Language) was developed to identify how markups, called tags, could be used to enhance text and graphics. The SGML standard doesn’t specify tag names or use, it just specifies rules for how such tags will work. This permits subset languages to develop tag names and functions appropriate to their objective. For example, popular markup languages used in Web pages are HTML, DHTML, and XML… I’m sure that you’ve heard of atleast two of these. The strength of any markup language is its ease of use for the user.
BUT ease of use does not translate to readability. YAML is a human friendly data serialization standard for all programming languages. So YAML is similar in a lot of ways to json. It also stores the data in custom { key : value } pairs which can be easily read. has steadily increased in popularity over the last few years. It’s often used as a format for configuration files, but its object serialization abilities make it a viable replacement for languages like JSON. For example, if you were creating a CV, it’d probably be something like:
---
Name: "Arka"
Address: "India"
Phone: 100
WorkFromHome: true
Mail: "[email protected]"
Internships:
- JournalDev
- MNO
- ABC
- XYZ
Education:
MSc: 7.6
BSc: 8.1
XII: 9
Courses:
Udemy: 15
Coursera: 3
References: "Mr. QWERTY, XYZ"
Let’s break this down:
The file starts with three dashes. These dashes indicate the start of a new YAML document. YAML supports multiple documents, and compliant parsers will recognize each set of dashes as the beginning of a new one. So you could write multiple documents within the same file.
The file has 5 pairs of k:v pairs. Name, Address and Email are string types, Phone is a big-int and WorkFromHome is a boolean.
Internships is an array containing 4 elements. Education is a dictionary with key:value pairs of its own. YAML supports nesting of as many dictionaries as you want. JSON and YAML have similar capabilities, and you can convert most documents between the formats.
YAML support in Golang
Go has three highly capable libraries for the encoding and decoding of yaml files:
- go-gypsy
- go-yaml
- goccy-yaml
Let’s check out each one.
1. go-gypsy
import "github.com/kylelemons/go-gypsy/yaml"
Gypsy is a simplified YAML parser written in Go. It is intended to be used as a simple configuration file.
Hyphens denote lists, and k:v pairs denote mappings:
1. map inside list:
- name: John Smith
age: 42
- name: Jane Smith
age: 45
2. list in map:
Scene:
- Meadow
- Forest
- Shady
Theme:
- Art
- Commercial
3. list of list:
- - one
- two
- three
- - un
- deux
- trois
- - ichi
- ni
- san
4. map of maps:
google:
company: Google, Inc.
ticker: GOOG
url: http://google.com/
yahoo:
company: Yahoo, Inc.
ticker: YHOO
url: http://yahoo.com/
2. go-yaml
The defacto standard library for YAML processing Go, go- yaml s based on a pure Go port of the well-known libyaml C library to parse and generate YAML data quickly and reliably.
First install using:
go get gopkg.in/yaml.v2
Then:
import "gopkg.in/yaml.v2"
3. goccy-yaml
This is an optional modification on the go-yaml package:
- Pretty format for error notifications
- Support Scanner or Lexer or Parser as public API
- Support Anchor and Alias to Marshaler
- Allow referencing elements declared in another file via anchors
- Extract value or AST by YAMLPath ( YAMLPath is like a JSONPath )
go get -u github.com/goccy/go-yaml
Parsing YAML File in Go
Create a file your_name.yml with your details. I made mine:
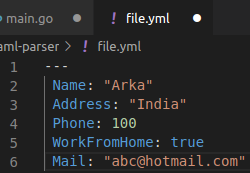
Then create a main.go file in the same directory, and use your filename and field names:
package main
import (
"fmt"
"io/ioutil"
"log"
"gopkg.in/yaml.v2"
)
type instanceConfig struct {
Name string `yaml:"Name"`
Address string `yaml:"Address"`
Phone int `yaml:"Phone"`
WFH bool
}
func (c *instanceConfig) Parse(data []byte) error {
return yaml.Unmarshal(data, c)
}
func main() {
data, err := ioutil.ReadFile("file.yml")
if err != nil {
log.Fatal(err)
}
var config instanceConfig
if err := config.Parse(data); err != nil {
log.Fatal(err)
}
fmt.Printf("%+v\n", config)
}
Then, if we go run go main.go file.yml, the output is as follows:

Neat !
References
- https://github.com/kylelemons/go-gypsy
- https://github.com/go-yaml/yaml
- https://github.com/topics/yaml-configuration