Packages allow us to focus on the code reuse principle aka the DRY (Don’t Repeat Yourself) principle. It enables us to write concise code in a structured manner. In this post, we are going to take a look at the package system in Go.
What is a package in Go?
A package is essentially a container of source code for some specific purpose. For example, the io package contains the code for the input-output system, while the fmt package handles the formatting of string and logging to console.
Importing a package
To use a package we must import one. Go throws error when we import package but don’t use it. So, be cautious about it otherwise we have to use the blank identifier.
Here is how we import packages.
import "packagename" // single package
import ( // multiple packages
"io"
"os"
)
Package aliasing and ignored import
A package when importing can be aliased as follows.
package main
import f "fmt"
// aliasing is when we use a different name for a package for our convenience
func main() {
f.Println("Hello") // Hello
}
The ignored import occurs when we put the blank identifier before the package import. The import but not used errors are ignored. Here is the way to do that.
package main
// import with blank identifier
import _ "fmt"
func main(){
// do nothing...
// throws no error regarding unused import
}
Package exported and non-exported member
A package’s items are only accessible if they are exported properly. Otherwise, it is private and can only be accessed inside it.
To export an item we simply make its first letter capital. That’s the rule to follow when making exported members in a package.
package hello
var Hello := "Hello World" // capital-first means public and exported
var bye := "See ya all" // small-first means private and not exported
Package nesting
Packages can be nested to an arbitrary depth. That means a single package can contain multiple sub-packages.
packageone/
|--packagetwo/
|--packagethree/
|--packagefour/
Creating a custom package
Creating a custom package is an easy task. We need to be inside the GOPATH and then create the directory for our custom package. Below is an example of a really basic custom package.
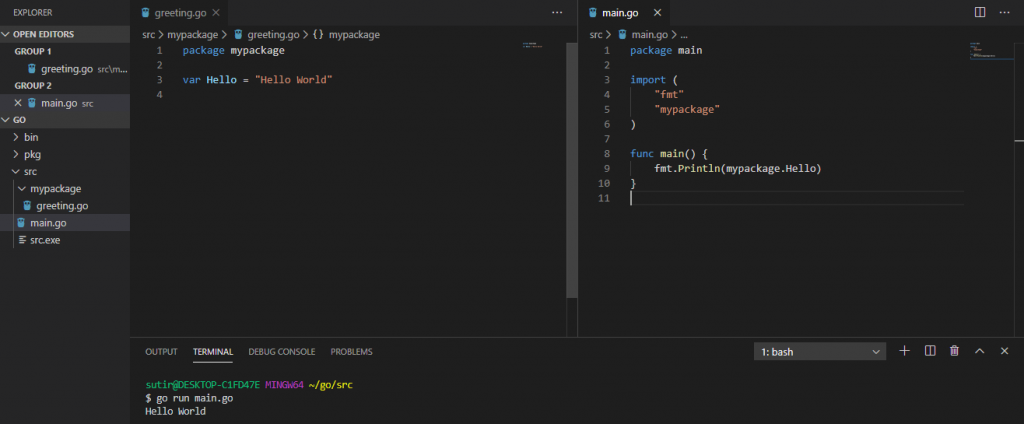
In the image above, it can be seen that the package is created with really basic code. The package contains a single file greeting.go which exports a member Hello. Then in our program, we use it after importing the mypackage.