The time package contains everything useful about time. In this post, we are going to explore the time package in Go.
The Sleep() function in time package
First, we need to import the time package to do something with it.
import "time"
The time package has a sleep function that can pause the main Go thread.
package main
import (
"fmt"
"time"
)
func main() {
fmt.Println(time.Now()) // 2020-02-25 23:00:00 +0000 UTC m=+0.000000001
time.Sleep(2 * time.Second)
fmt.Println(time.Now()) // 2020-02-25 23:00:02 +0000 UTC m=+2.000000001
}
The types inside the time package
The package contains multiple types that are useful in many different situations. Here are the important ones.
1. The Duration type represents the passed time or the time interval in Go.
package main
import (
"fmt"
"time"
)
func main() {
t1 := time.Now()
time.Sleep(2 * time.Second)
t2 := time.Now()
fmt.Println("Duration was: ", t2.Sub(t1)) // Duration was: 2s
}
The Duration can produce up to nanoseconds precision. To get those properties we need to call the type from Duration object.
package main
import (
"fmt"
"time"
)
func main() {
t1 := time.Now()
time.Sleep(3 * time.Second)
t2 := time.Now()
t := t2.Sub(t1)
fmt.Println(t, t.Milliseconds(), t.Microseconds(), t.Nanoseconds()) // 3s 3000 3000000 3000000000
}
2. The Location represents the time zone specific location.
3. The Month type represents the month as an int.
4. The Time type represents the time and contains many functions for different operations as we will see.
The time functions
The time struct has many functions associated with it. Here are some of them.
package main
import (
"fmt"
"time"
)
func main() {
var t time.Time
t = time.Now()
fmt.Println(t.Date())
fmt.Println(t.Day())
fmt.Println(t.Month())
fmt.Println(t.Second())
fmt.Println(t.Minute())
}
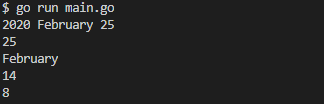
You can get the timezone using the handy function Zone().
package main
import (
"fmt"
"time"
)
func main() {
var t time.Time
t = time.Now()
fmt.Println(t.Zone()) // IST 19800
}
We can add or subtract time using the functions and then precisely output the time.
The Unixnano function outputs the Unix time which is in nanoseconds.
package main
import (
"fmt"
"time"
)
func main() {
var t time.Time = time.Now()
fmt.Println(t.UnixNano()) // 1257894000000000000
}
The Before() and After() functions in time
The before and after functions check whether a time is before or after another time.
package main
import (
"fmt"
"time"
)
func main() {
t1 := time.Date(2017, 1, 1, 0, 0, 0, 0, time.UTC)
t2 := time.Date(2019, 2, 1, 0, 0, 0, 0, time.UTC)
fmt.Println(t1.Before(t2)) // true
fmt.Println(t1.After(t2)) // false
}