Go has a built-in package for reading and writing CSV files. This post will cover the necessary details for working with CSV files in Golang.
What is a CSV File?
CSV is an encoding which stands for Comma Separated Values. This encoding delimits the data using commas. It is one of the most used encodings to date. Go has encoding/csv
package to handle the CSV data.
Importing encoding/csv package
To use CSV with Go we need to import the encoding/csv
package as shown below.
import "encoding/csv"
We will also import the os package which will help us open or create files on the go.
import "os"
Reading a CSV file in Golang
Now, we will try reading a CSV file. To do that, we first need to open it using the os package. Then we create a CSV reader from that file and read its content. Here is the code on how to read a CSV file in Go.
package main
import (
"encoding/csv"
"fmt"
"os"
)
func main() {
file, err := os.Open("testcsv.csv")
if err != nil {
fmt.Println(err)
}
reader := csv.NewReader(file)
records, _ := reader.ReadAll()
fmt.Println(records)
}
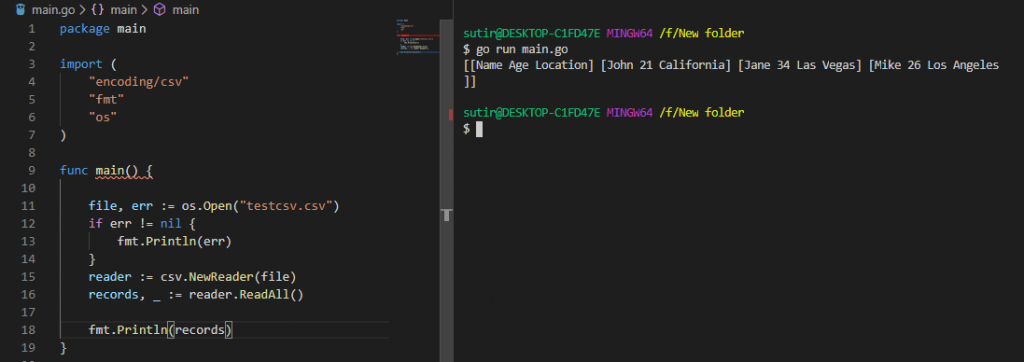
As can be seen, the reader reads a predefined CSV file and outputs the data to the console.
Writing a CSV file in Golang
We will be able to write a CSV file in the same way we read the file. First, we need to create the file for writing using the os package.
package main
import (
"encoding/csv"
"fmt"
"os"
)
func main() {
f, e := os.Create("./People.csv")
if e != nil {
fmt.Println(e)
}
writer := csv.NewWriter(f)
var data = [][]string{
{"Name", "Age", "Occupation"},
{"Sally", "22", "Nurse"},
{"Joe", "43", "Sportsman"},
{"Louis", "39", "Author"},
}
e = writer.WriteAll(data)
if e != nil {
fmt.Println(e)
}
}
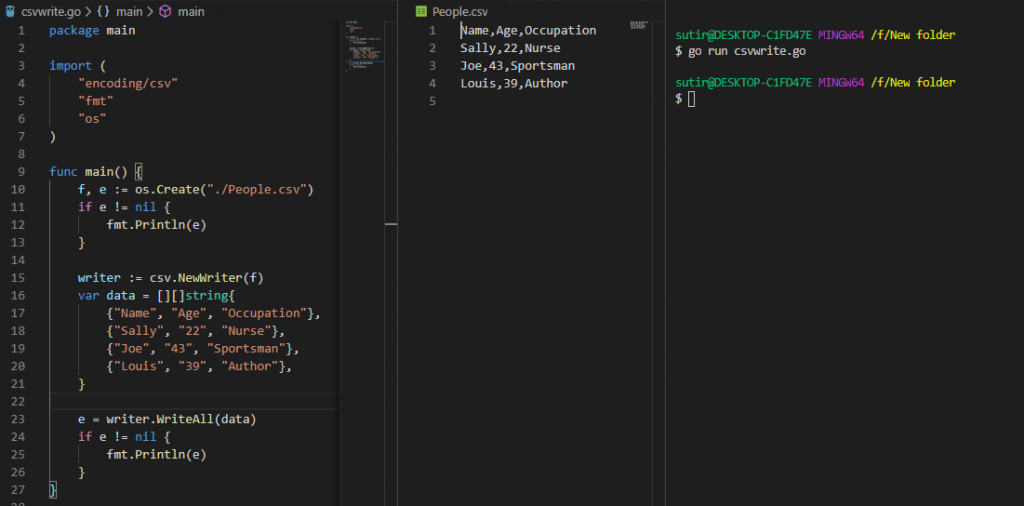
In the code above the slice of the strings is taken as data and written into the CSV file using the writer obtained.