In this post, we are going to explore how to write into files in Golang. Go has the io, os, and ioutil (deprecated in Go1.16) package which makes writing a file a lot easier. So, let’s see what are the different ways we can do it.
First step: Creating a File
In order to write something inside a file, we must create the file for writing. Here are the steps necessary to create a file.
f, err := os.Create("filename.ext") // creates a file at current directory
if err != nil {
fmt.Println(err)
}
Also, we don’t want to forget to close that file. So, immediately using defer to close the file is an idiomatic way in Go.
defer f.Close()
Write strings in a file
Here are some of the ways to write strings in a file.
1. Using the ioutil package (Deprecated in Go1.16)
The ioutil package has a function called WriteFile, which can be used to directly write some strings in a file without much effort. It will be converted to a byte slice and then written inside the file. Here is an example showing that. In this function, we need to insert the file mode as well. We will put 0644 for it.
package main
import (
"io/ioutil"
)
func main() {
s := []byte("This is a string") // convert string to byte slice
ioutil.WriteFile("testfile.txt", s, 0644) // the 0644 is octal representation of the filemode
}
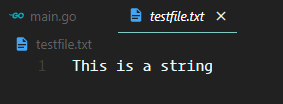
Since this package has been deprecated, I would recommend you use the “os” or “io” package.
2. Using a more conventional approach using the os package
The style to follow here is as follows:
- Create a file for writing.
- Close it immediately using
defer
so that it gets closed after all the operations are done on it. - Write inside it
Here is an example showing how it’s done.
package main
import (
"fmt"
"os"
//"io/ioutil"
)
func main() {
// create the file
f, err := os.Create("test.txt")
if err != nil {
fmt.Println(err)
}
// close the file with defer
defer f.Close()
// do operations
//write directly into file
f.Write([]byte("a string"))
// write a string
f.WriteString("\nThis is a pretty long string")
// // write from a specific offset
f.WriteAt([]byte("another string"), 42) // 12 is the offset from start
}
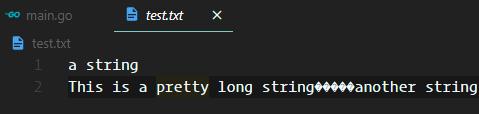
In the code above, the write function writes into the file directly.
The WriteString function does exactly what it says, writes a string into a file.
The WriteAt function takes two arguments, the string converted into bytes array and an offset. The function writes the string at that offset in that file.
3. Writing to a File using the io package
We can use io.WriteString() function to write a string into a file. Here is the simple code to achieve it.
package main
import (
"fmt"
"io"
"os"
)
func main() {
// create the file
f, err := os.Create("test_io.txt")
if err != nil {
fmt.Println(err)
}
// close the file with defer
defer f.Close()
// write a string
io.WriteString(f, "This is a long string")
}
4. Append to an existing file
Appending to an existing file is simple. We open the file and then append using the Fprintln function.
package main
import (
"fmt"
"os"
)
func main() {
f, err := os.OpenFile("test.txt", os.O_APPEND|os.O_WRONLY, 0644)
if err != nil {
fmt.Println(err)
return
}
newLine := "This is a string which will be appended."
_, err = fmt.Fprintln(f, newLine)
if err != nil {
fmt.Println(err)
}
}
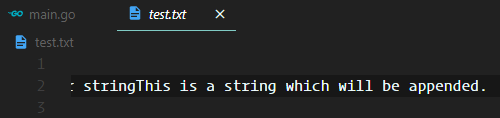
These are some of the ways file writing operations are handled in Go.