REST API is by far the most used form of API in the world. This post will focus on creating a simple REST API in Golang.
What is the REST API?
REST stands for Representational State Transfer. It is a way clients connect to servers to get data. The server serves the data via HTTP/HTTPS. The client access specific endpoints to get data, delete data, modify and do many other things in the server.
Here are some examples of how REST API looks like.
https://jsonplaceholder.typicode.com/todos/1
When we hit that endpoint we get:
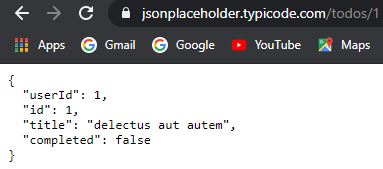
As can be seen, the JSON data is being served via that URL.
Creating our REST API
Now, we will create a simple REST API that will serve JSON data via endpoints.
Required imports
For this tutorial, we need to import the json and the HTTP package.
import (
"encoding/json"
"net/http"
)
Create a data structure
First, we need to create the structure of data we are going to serve via the API. Below is the structure we are going to serve.
type Post struct {
Title string `json:"Title"`
Author string `json:"Author"`
Text string `json:"Text"`
}
Setting up HTTP handler
Then we need to set up a handler that will handle the API endpoint. Here the handler has the array of data that we are going to serve.
func PostsHandler(w http.ResponseWriter, r *http.Request) {
posts := []Post{
Post{"Post one", "John", "This is first post."},
Post{"Post two", "Jane", "This is second post."},
Post{"Post three", "John", "This is another post."},
}
json.NewEncoder(w).Encode(posts)
}
The posts array will be served as json as the next line encodes the data.
Now, we will set the endpoint up along with the handler.
http.HandleFunc("/posts", PostsHandler)
Then we serve the endpoint via a port.
http.ListenAndServe(":5051", nil)
Now, if we open up the endpoint in our browser, we will get the JSON data served.
The output will be like this as shown below. Here I am using an extension for chrome to get the formatted output.
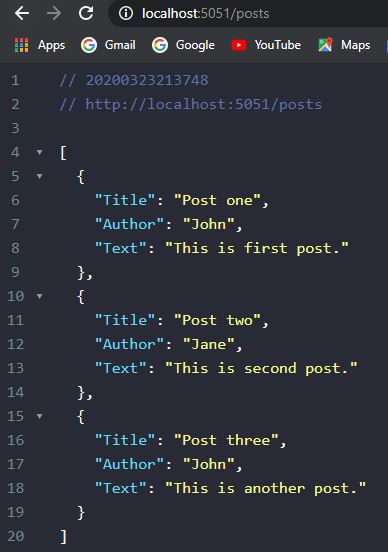
The final code
The full code is as follows:
package main
import (
"encoding/json"
"net/http"
)
type Post struct {
Title string `json:"Title"`
Author string `json:"Author"`
Text string `json:"Text"`
}
func PostsHandler(w http.ResponseWriter, r *http.Request) {
posts := []Post{
Post{"Post one", "John", "This is first post."},
Post{"Post two", "Jane", "This is second post."},
Post{"Post three", "John", "This is another post."},
}
json.NewEncoder(w).Encode(posts)
}
func main() {
http.HandleFunc("/posts", PostsHandler)
http.ListenAndServe(":5051", nil)
}
Use of REST API
REST API is primarily used to serve content via an endpoint. It creates a way to provide data to clients from the servers. Each specific endpoints provide specific data required. This creates modularize data serving and prevents data wastage.