Creating HTTP services in Go is not complex. The HTTP package saves us a lot of time when doing so. In this post, we will work with the HTTP package to create an HTTP server in Go.
Required imports
To work with HTTP we need to import the HTTP package. It contains client and server implementation for HTTP.
import "net/http"
Creating a basic HTTP Server in Golang
To create a basic HTTP server, we need to create an endpoint. In Go, we need to use handler functions that will handle different routes when accessed. Here is a simple server that listens to port 5050.
package main
import (
"fmt"
"net/http"
)
func main() {
// handle route using handler function
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Welcome to new server!")
})
// listen to port
http.ListenAndServe(":5050", nil)
}
Now, when we access the localhost:5050 we get the string shown in the browser as a response.
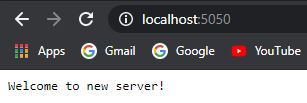
The server is running!
Handling different routes
We can handle different routes just like before. We can simply use different handlers for each route we want to handle. Here are some examples.
package main
import (
"fmt"
"net/http"
)
func main() {
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Welcome to new server!")
})
http.HandleFunc("/students", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Welcome Students!")
})
http.HandleFunc("/teachers", func(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Welcome teachers!")
})
http.ListenAndServe(":5050", nil)
}
Here we have added different routes. Now when we access them we get different results.
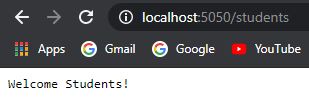
When we access the teachers’ route we get different results.
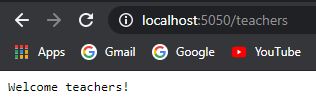
It can be seen, that each route is handled by different handlers.
Using a server mux
A mux is a multiplexer. Go has a type servemux defined in http package which is a request multiplexer. Here’s how we can use it for different paths. Here we are using the io package to send results.
package main
import (
"io"
"net/http"
)
func welcome(w http.ResponseWriter, r *http.Request) {
io.WriteString(w, "Welcome!")
}
func main() {
mux := http.NewServeMux()
mux.HandleFunc("/welcome", welcome)
http.ListenAndServe(":5050", mux)
}
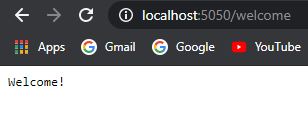