XML is a data-interchange format. It is heavily used alongside with JSON. Working with XML is simple in Golang. In this post, we will see how to work with XML in Golang.
Required imports
To work with XML we need to import encoding/xml package.
import "encoding/xml"
Encoding with XML
Now, we can start working with XML in Go. First, we need to define the XML format alongside the data structure. Then we can simply marshal or unmarshal depending on what we want to do.
Here is an example of encoding to XML data:
package main
import (
"encoding/xml"
"fmt"
)
type Person struct {
Name string
Age int
}
func main() {
p := &Person{"Jack", 22}
v, _ := xml.MarshalIndent(p, "", " ")
fmt.Println(string(v))
}
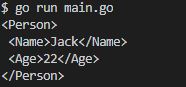
Decoding custom data to struct
We can unmarshal custom data to a struct using the XML package. Here is an example of a custom data unmarshalled to a struct.
package main
import (
"encoding/xml"
"fmt"
)
type Book struct {
Name string `xml:"Name"`
Author string `xml:"Author"`
}
func main() {
s := `
<Book>
<Name>War And Peace</Name>
<Author>Leo Tolstoy</Author>
</Book>
`
b := &Book{}
xml.Unmarshal([]byte(s), b)
fmt.Println(b)
}
Observe that, we declared the names of the tags beside the struct to declare what data goes to what. Also, when unmarshalling we use the string that uses backticks.
