Stings are always defined using characters or bytes. In Golang, strings are always made of bytes. Go uses UTF-8 encoding, so any valid character can be represented in Unicode code points.
What is Golang rune?
A character is defined using “code points” in Unicode. Go language introduced a new term for this code point called rune.
Go rune is also an alias of type int32 because Go uses UTF-8 encoding. Some interesting points about rune and strings.
- Strings are made of bytes and they can contain valid characters that can be represented using runes.
- We can use the rune() function to convert string to an array of runes.
- For ASCII characters, the rune value will be the same as the byte value.
Finding rune of a character in Go
Let’s look at a program to print rune of a character.
package main
import (
"fmt"
)
func main() {
s := 'a'
s_rune := rune(s)
fmt.Println(s_rune)
}
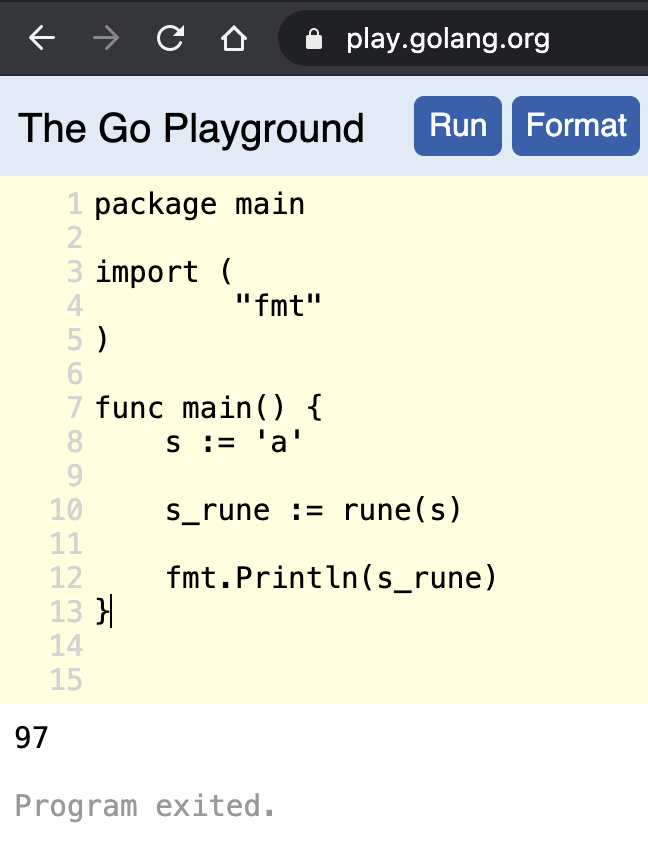
Golang String to rune
Let’s print the rune values of string characters using rune() function.
package main
import (
"fmt"
)
func main() {
s := "Golang"
s_rune := []rune(s)
fmt.Println(s_rune) // [71 111 76 97 110 103]
}
The integer array output looks same if we use the byte() function to convert into byte values array. So, what is the difference between byte and rune, let’s look into that in the next section.
Understanding difference between byte and rune
Let’s print byte array and rune of a string having non-ascii characters.
package main
import (
"fmt"
)
func main() {
s := "GÖ"
s_rune := []rune(s)
s_byte := []byte(s)
fmt.Println(s_rune) // [71 214]
fmt.Println(s_byte) // [71 195 150]
}
The special Unicode character Ö rune value is 214 but it’s taking two bytes for encoding.
Recommended Read: sleep in Go
Reference: GoDocs on Rune