In this post, we will see templates and their usages in the Go programming language.
What are templates?
Templates are a common view through which we can pass data to make that view meaningful. It can be customized in any way to get any output possible.
Template packages
Templating in Go comes with two packages text/template
and html/template
. The text package allows us to interpolate texts with the template, while HTML templating helps us by providing the safe HTML code.
Parts of a template
Let’s have a look at different parts of the code before we start using them.
1. Template actions
Template actions are the main control flows, data evaluations, functions. These actions control how the final output will show up. Here are some basic actions as shown below.
{{ /* a comment inside template */ }}
To render the root element:
{{ . }}
2. Control structures
Control structures determine the control flow of the template. It helps to produce structured output. Below are some control structures in templating.
The if statement:
{{ if .condition }} {{ else }} {{ end }}
The range block:
{{ range .Items }} {{ end }}
Range block iterates over the list provided.
3. Functions
Functions can be used inside templates as well. We can use the pipe ( | ) operator to use predefined functions.
Parsing templates in Go
Now we will parse some text and HTML templates.
1. Accessing data
To access the data passed we use the dot (.) syntax like shown below.
{{ .data }}
2. Parsing a text template
Now we will parse a text template as shown below.
package main
import (
"os"
"text/template"
)
type User struct {
Name string
Bio string
}
func main() {
u := User{"John", "a regular user"}
ut, err := template.New("users").Parse("The user is {{ .Name }} and he is {{ .Bio }}.")
if err != nil {
panic(err)
}
err = ut.Execute(os.Stdout, u)
if err != nil {
panic(err)
}
}
In the code above a struct is passed in a text template the output is shown below.

3. Parsing an HTML template
Let’s try parsing an HTML document. Below show codes are used for this example.
In file hello.gohtml
:
<h1>Go templates</h1>
<p>The user is {{ .Name }}</p>
<h2>Skills:</h2>
{{ range .Skills }}
<p>{{ . }}</p>
{{ end }}
In file main.go:
package main
import (
"os"
"html/template"
)
func main() {
t, err := template.ParseFiles("templates/hello.gohtml")
if err != nil {
panic(err)
}
data := struct {
Name string
Skills []string
}{
Name: "John Doe",
Skills: []string{
"C++",
"Java",
"Python",
},
}
err = t.Execute(os.Stdout, data)
if err != nil {
panic(err)
}
}
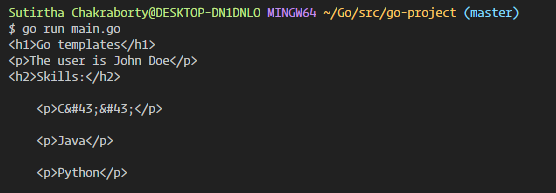
Template Verification in Go
To verify if a template is valid, we use template.Must()
function. It helps verify the template during parsing.
tmp := template.Must(template.ParseFiles("tmp.html"))
We use it like shown before.
Usage of Templating in Go Programming
Templating can be extremely useful in many conditions not just in general web page creation only. But,
- Generate a web page using data from API.
- Generate emails
- Create web sites using HUGO templating.