Building applications for different platforms is one of the most important things out there. Go has very strict policies on how it can be done. In this post, we are learn how to build a Golang application.
GOOS and GOARCH
The GOOS and GOARCH define the OS and architecture for current program. When we output the env we can see what they are set.
go env GOOS GOARCH

These are the platform parameters for which the go build command will work.
Golang Supported Platforms
To see all the possible platforms and builds supported by Go, here is a command that will list all of it.
go tool dist list
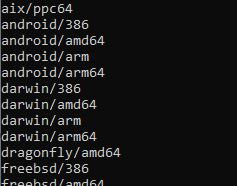
Building for a custom OS and Arch
To build for a custom OS and ARCH we need to use the build tags. Here are some examples. Say, we want to create a build for linux/386, we can do it by using a build tag like this.
// + build linux,386
// ...file source
It’s just a comment before a file.
Now, if we run go build it will take this file into compilation only when the build tag matches.
Here is an example:
In a file include.go contains an int that will only be taken for compilation if the platform is not windows. Now, what happens if we use it in main.go file.
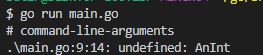
Since the build tag for the include file is:
// +build !windows
The code for the files are:
// main.go contents
package main
import (
"fmt"
)
func main() {
fmt.Println("Hello world")
fmt.Println(AnInt)
}
// include.go contents
// +build !windows
package main
var AnInt = 42
It won’t take this include file for windows compilation.
Now, we can create a windows specific file to build for it including that integer or anything else. The build system allows for modularized builds for different platforms and architectures that can be easily done without much hassle. This is where the build tool shines.
Recommended: Go Makefiles