MongoDB is one of the most used NoSQL database out there. It is also a very new database. In this post, we are going to use MongoDB alongside Golang to create CRUD system.
Installing MongoDB
To download MongoDB head over to mongodb.com and hover over the software menu and click on the community server and then follow accordingly to download the server suitable for your OS.
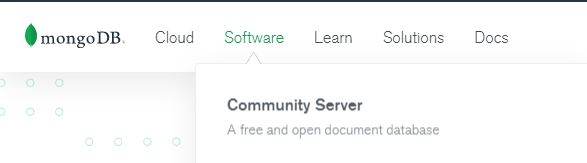
Now, to check if installation was correct here is the command that shows installation version:
mongo --version
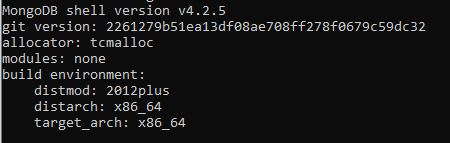
Starting MongoDB Server
We need to start MongoDB server before using it. The command is mongod. The server will start on port 27017. Now, we can run the CLI tool to connect to the server and start working.
mongod // starts server
mongo // starts CLI
After starting the CLI tool we will get a prompt “>” in which we can enter the commands to interact with the databases. Here are some commands to try out.
show dbs // it will show all databases available with sizes
use <dbname> // will use the <dbname> database, will create if none exists
Connect to the MongoDB Server using Go
Here is the required imports:
"go.mongodb.org/mongo-driver/bson"
"go.mongodb.org/mongo-driver/mongo"
"go.mongodb.org/mongo-driver/mongo/options"
To do database operations first, we need to connect to the server. To do that, here is the code.
// Set client options
clientOptions := options.Client().ApplyURI("mongodb://localhost:27017")
// Connect to MongoDB
client, e := mongo.Connect(context.TODO(), clientOptions)
CheckError(e)
// Check the connection
e = client.Ping(context.TODO(), nil)
CheckError(e)
// get collection as ref
collection := client.Database("testdb").Collection("people")
1. Golang MongoDB Inserting Record
To insert data we first declare data struct that can be used to provide the structure of the data we are going to use.
type Person struct {
Name string
Age int
}
Then we can use the data in a structured manner to store it:
// insert
john := Person{"John", 24}
jane := Person{"Jane", 27}
ben := Person{"Ben", 16}
_, e = collection.InsertOne(context.TODO(), john)
CheckError(e)
persons := []interface{}{jane, ben}
_, e = collection.InsertMany(context.TODO(), persons)
CheckError(e)
From the CLI, we can check if the data is inserted using the command shown below. In order to use it, we also need to be using the database as well. Use “use dbname” to use the database and then enter the following command:
db.collectionName.find()

2. Updating data in MongoDB
To update existing data we will use the bson filters. This the way we can find the data and change it whenever needed. Below is an example showing how to use bson structure for filters.
// update
filter := bson.D{{"name", "John"}}
update := bson.D{
{"$set", bson.D{
{"age", 26},
}},
}
_, e = collection.UpdateOne(context.TODO(), filter, update)
CheckError(e)

3. Finding data in MongoDB Collection
Finding data is also done using the bson filters. Here is a simple example illustrating the way to use it.
var res Person
e = collection.FindOne(context.TODO(), filter).Decode(&res)
fmt.Println(res)
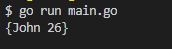
4. Delete MongoDB Record using Golang
Deleting data is also easier and can be done using the bson filters. Here is an example that deletes all data.
// delete
_, e = collection.DeleteMany(context.TODO(), bson.D{{}})
CheckError(e)

Golang MongoDB CRUD Example – Complete Code
Here is the full program of using mongodb with Golang.
package main
import (
"context"
"fmt"
"go.mongodb.org/mongo-driver/bson"
"go.mongodb.org/mongo-driver/mongo"
"go.mongodb.org/mongo-driver/mongo/options"
)
type Person struct {
Name string
Age int
}
func main() {
// Set client options
clientOptions := options.Client().ApplyURI("mongodb://localhost:27017")
// Connect to MongoDB
client, e := mongo.Connect(context.TODO(), clientOptions)
CheckError(e)
// Check the connection
e = client.Ping(context.TODO(), nil)
CheckError(e)
// get collection as ref
collection := client.Database("testdb").Collection("people")
// insert
john := Person{"John", 24}
jane := Person{"Jane", 27}
ben := Person{"Ben", 16}
_, e = collection.InsertOne(context.TODO(), john)
CheckError(e)
persons := []interface{}{jane, ben}
_, e = collection.InsertMany(context.TODO(), persons)
CheckError(e)
// update
filter := bson.D{{"name", "John"}}
update := bson.D{
{"$set", bson.D{
{"age", 26},
}},
}
_, e = collection.UpdateOne(context.TODO(), filter, update)
CheckError(e)
// find
var res Person
e = collection.FindOne(context.TODO(), filter).Decode(&res)
fmt.Println(res)
// delete
_, e = collection.DeleteMany(context.TODO(), bson.D{{}})
CheckError(e)
}
func CheckError(e error) {
if e != nil {
fmt.Println(e)
}
}