Go has a very flexible module system. From version 1.11 Go supports the use of modules. In this post, we are going to create a simple module in Go.
What is a Go Module?
A module is a collection of packages in a versioned manner. A go module contains go.mod file in the root directory.
Go modules help manage version changes of packages really easy with its dependency management system. That’s why learning how to create a go module is essential.
Creating a Go Module
Now, we will create an example Go module. We will call it my-module.
First, we need to create a folder inside the GOPATH src directory.
Then we for simplicity’s sake add two files. One is general go code and the other is a testing file.
// mymod.go
package hello
func GetModName() string {
return "My module"
}
// mymod_test.go
package hello
import "testing"
func TestHello(t *testing.T) {
want := "My module"
if got := GetModName(); got != want {
t.Errorf("Hello() = %q, want %q", got, want)
}
}
Now, we run go test to see the result.
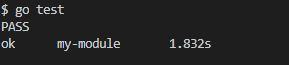
Now, we need to have a go.mod file in that directory. That will allow us to manage dependency for this module.
To do it, run this command.
go mod init
After running this command we will get a go.mod file, that will look as below:
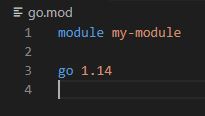
Now, currently, we don’t have dependencies. But in any case, we add it the mod file will get updated.
Now, suppose we add the logrus package as a dependency then after adding the package we will run the go test command again.
Now, the file will look like as follows:
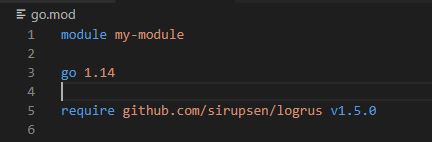
As can be seen, it got updated with the package and version name.
This is how modules simplify the package and dependency management in Go.
Now, to get all the dependency my package has, we run the following command:
go list -m all
The output is for my case as follows:
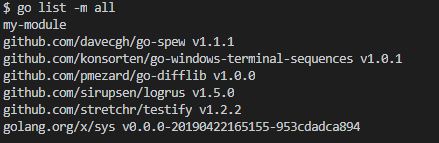
Now, whenever we update its dependencies and run the test it will automatically fetch those packages without anything from our side. This is what makes modularization a very powerful tool in Golang.