Hello again! While the previous few tutorials were mostly based on simple concepts, we’ll be now looking at a more practical example – Building a custom-made Slack bot using Go!
We’ll be now creating our own Slack bot which can push messages to a channel, notifying all users who are subscribed to it.
Let’s get started!
Setup Slack Tokens
Before we proceed with the main program, we need to do some setup.
We first need to create a new Bot user on Slack. To do this on slack, you need to create a new Slack App and create your new Bot user.
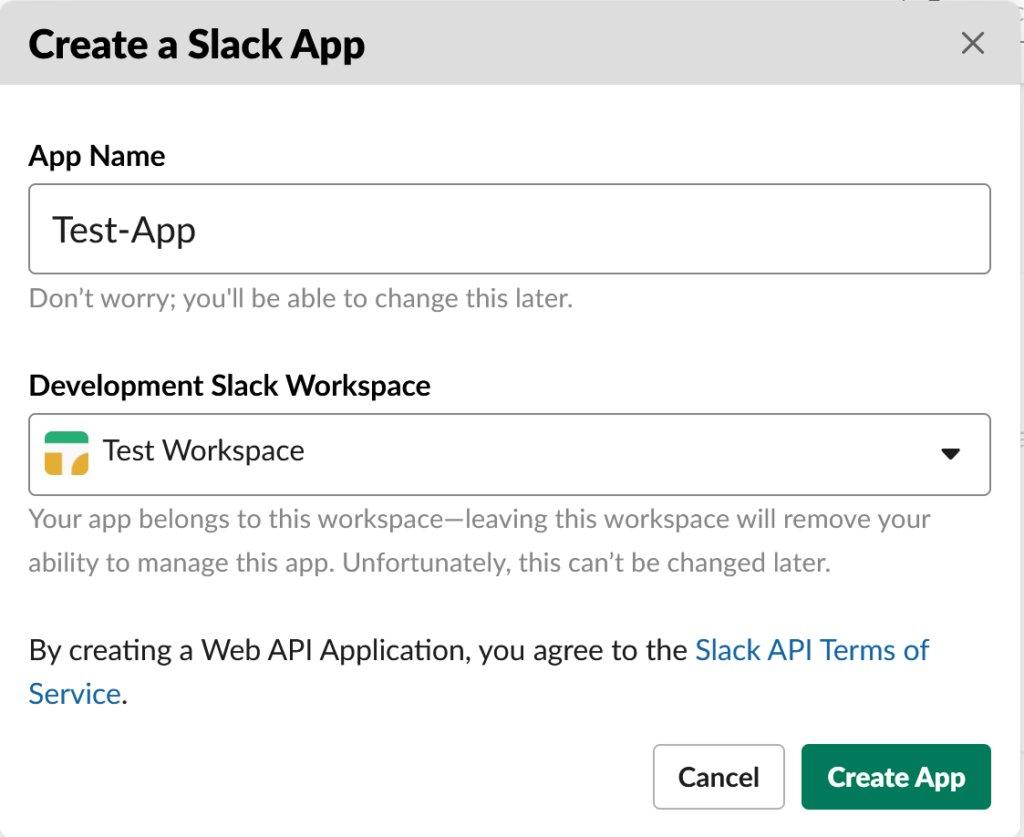
After you create the app, you can select the option for generating an OAuth token for your bot user.
You’ll need to add some OAuth scopes for this – Since our bot now only needs to read and post messages on channels, we’ll use the scopes in the below image.
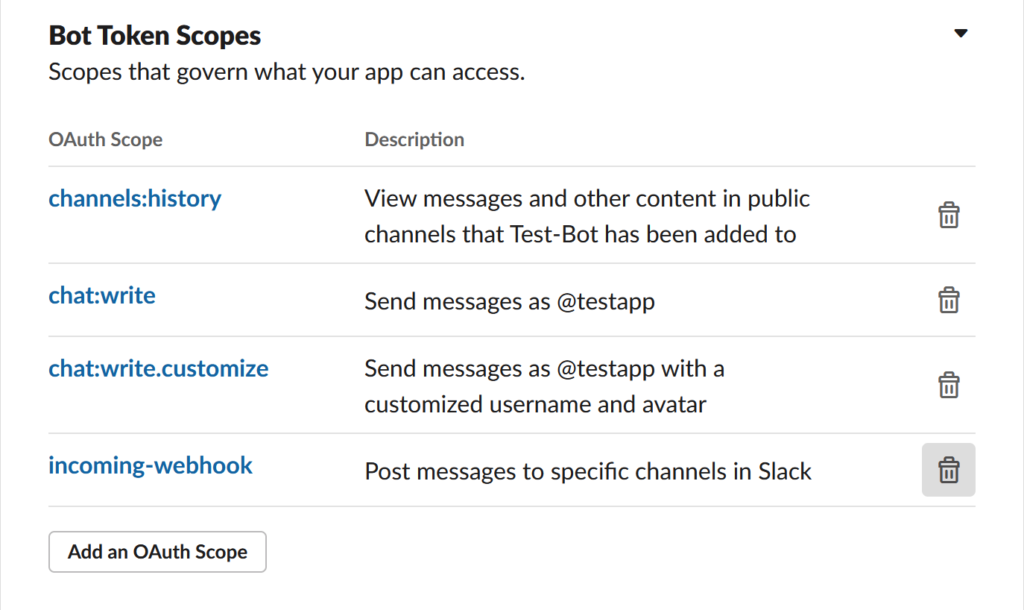
However, feel free to customize it as per your needs!
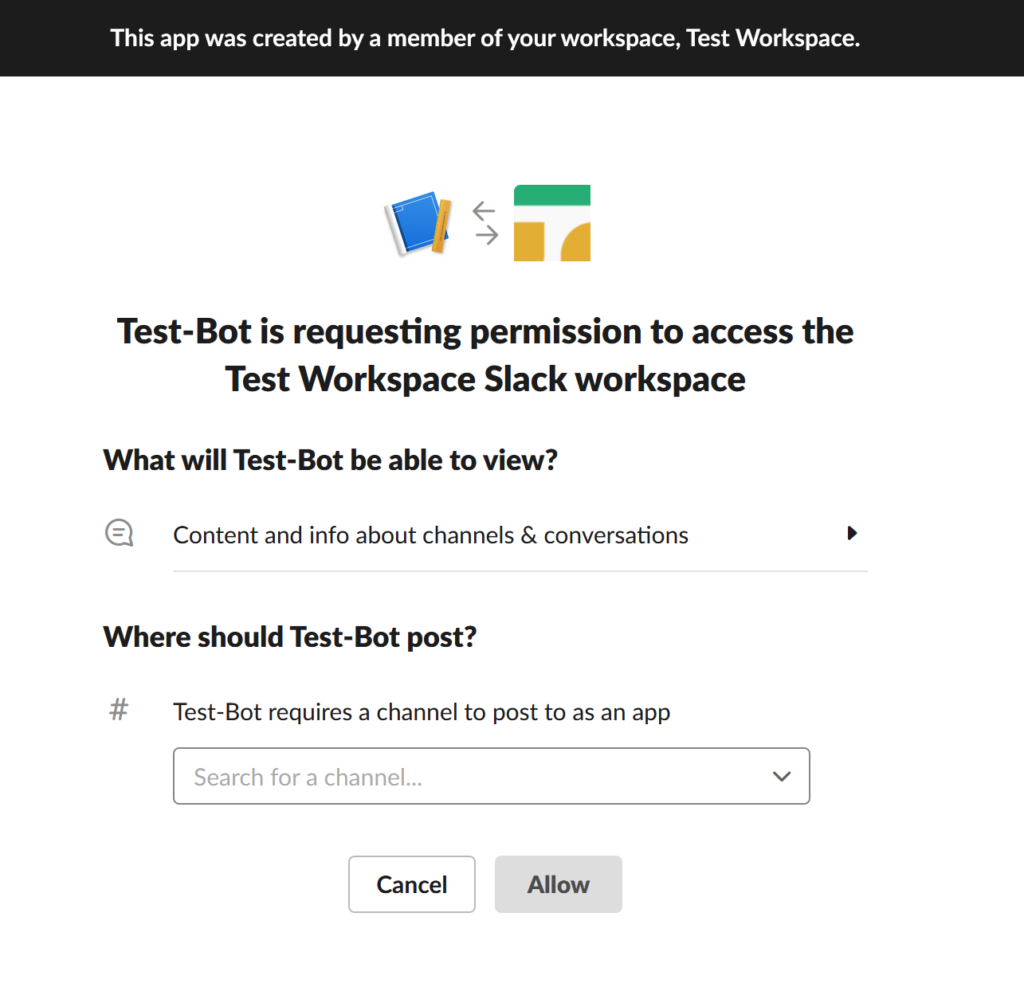
Once we authorize the use of this OAuth token on our workspace, we can (finally) start integrating with the Go client API.
Install the Go Package for Slack
We’ll be using the slack-go
package for the client. To install this, we’ll use go get
:
go get -u github.com/slack-go/slack
Building our Slack Bot in Golang
We’ll have the bot post messages in a new channel within our workspace.
To do this, we need to use a CHANNEL_ID
for this new channel.
To get the channel ID, open the slack workspace from your browser and go to the new channel. The URL must be of this format:
https://app.slack.com/client/xxxxxxxx/CHANNEL_ID/details/top
Copy the CHANNEL_ID
part of the URL.
package main
import (
"log"
"github.com/slack-go/slack"
)
func main() {
OAUTH_TOKEN := "OAUTH_TOKEN" // Paste your bot user token here
CHANNEL_ID := "CHANNEL_ID" // Paste your channel id here
api := slack.New(OAUTH_TOKEN)
attachment := slack.Attachment{
Pretext: "Pretext",
Text: "Hello from GolangDocs!",
}
channelId, timestamp, err := api.PostMessage(
CHANNEL_ID,
slack.MsgOptionText("This is the main message", false),
slack.MsgOptionAttachments(attachment),
slack.MsgOptionAsUser(true),
)
if err != nil {
log.Fatalf("%s\n", err)
}
log.Printf("Message successfully sent to Channel %s at %s\n", channelId, timestamp)
}
Finally, before you start your go program, we must explicitly invite our Slack bot to this channel. Otherwise, you’ll get an error similar to this:
Error: not_in_channel
This indicates that the bot is still not there in the channel, so we need to invite the bot/app.
We can do it using /invite @YOUR_APP_NAME
Slack Command in the Slack GUI.
After you invite the bot, you can run the program and see the results!
Sample Output
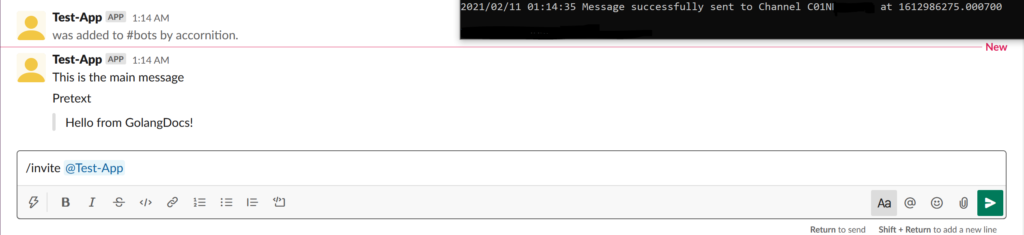
Once you finally get this bot up and running, you can explore various other things, such as giving regular updates from an API endpoint or actually interacting with real users via commands.
Until next time!