Good morning, Gophers! Today I bring to you another new fascinating package I’ve discovered, and which I’ve been tinkering with for a while now. And that is the finance-go package created by Michael Ackley, which allows us to directly retrieve stock market data on various crypto-currencies and quotes, options, price charts, etc. pulled from Yahoo Finance.
This go package aims to provide a go application with access to current and historical financial markets data in streamlined, well-formatted structures.
finance-go, GitHub
It provides the following features:
- Detailed realtime quotes across most instruments (minus fixed-income)
- Lists of OHLCV quotes for various time frames and aggregations
- Equity options series for most expirations
Installing finance-go package
It is very simple to install this package using the terminal:
go get github.com/piquette/finance-go
Getting stock quote details using finance-go
Let’s create a new file main.go, and import our necessary packages:
package main
import (
"flag"
"fmt"
"os"
"github.com/piquette/finance-go/quote"
"github.com/sirupsen/logrus"
)
The logrus package has a very handy formatting option that allows us to use log.Fatal as if it was a printf function.
We shall be using direct terminal inputs, hence the os package.
Then for the os input, using flag.Parse and os.Args:
func main() {
flag.Parse()
if len(flag.Args()) == 0 {
logrus.Fatalf("No argument given. Please input one stock symbol. Example: %v CLDR", os.Args[0])
}
We define the stock symbol as a variable smbl. Then we use the quote package to connect with Yahoo Finance to get the details of the stock in a custom struct called Finance.quote:
smbl := flag.Args()[0]
q, _ := quote.Get(smbl)
and finally we print all the valuable information we want like name, highs and lows of the price, etc:
fmt.Printf("------- %v -------\n", q.ShortName)
fmt.Printf("Current Price: $%v\n", q.Ask)
fmt.Printf("52wk High: $%v\n", q.FiftyTwoWeekHigh)
fmt.Printf("52wk Low: $%v\n", q.FiftyTwoWeekLow)
}
If we go run main.go this, we’ll get an error.
We need to enter an argument, so here are a few examples:
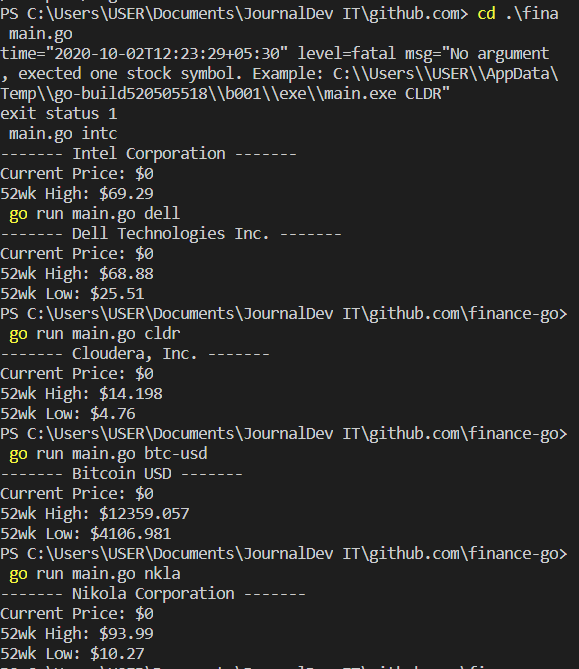
I’m not exactly sure why the current price is not fetching, but it is a yahoo problem and not due to our code.
Go to the Yahoo Finance page and search for your favorite stock, and using the name, do the search.
As a last part, this is fine. But typing each stock symbol individually is a lot of work. Instead we can just iterate over the whole list:
func main() {
flag.Parse()
if len(flag.Args()) == 0 {
logrus.Fatalf("No argument provided, exected at least one stock symbol. Example: %v cldr goog aapl intc amd ...", os.Args[0])
}
cf := accounting.Accounting{Symbol: "$", Precision: 2}
smbls := flag.Args()
iter := quote.List(smbls)
for iter.Next() {
q := iter.Quote()
fmt.Printf("------- %v -------\n", q.ShortName)
fmt.Printf("Current Price: %v\n", cf.FormatMoney(q.Ask))
fmt.Printf("52wk High: %v\n", cf.FormatMoney(q.FiftyTwoWeekHigh))
fmt.Printf("52wk Low: %v\n", cf.FormatMoney(q.FiftyTwoWeekLow))
fmt.Printf("-----------------\n")
}
}
Also, you may need to import this if the editor doesn’t for you:
"github.com/leekchan/accounting"
Now you can simply call all of them at the same time:
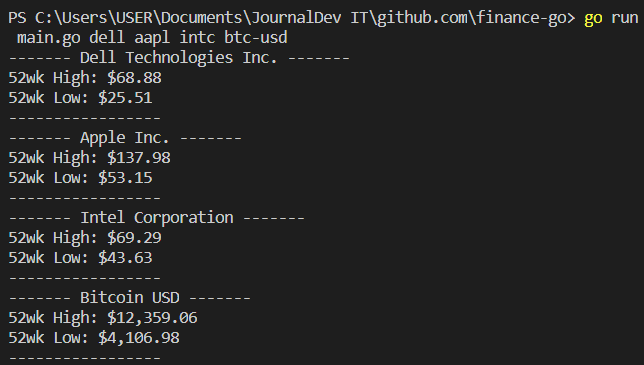
Go qtrn CLI tool
Now, the finance-go package itself requires some degree of financial knowledge, which, I’ll admit I am not an expert. However, the kind open-source developers for the Go language have created a CLI (Command Line Interface) tool for making financial markets analysis. According to their Github page,
This project is intended as a living example of the capabilities of the finance-go library.
It is easily installed with:
go get github.com/piquette/qtrn
and that’s it !
You now have a fully functioning finance terminal. It supports various methods such as write ( which can write the data from Yahoo Finance directly into a CSV file on your computer ), and chart ( which can create a chart of the company stock prices within the terminal ).
For example, if we continue with AAPL (Apple Inc.), then the command:
qtrn quote AAPL
gives:
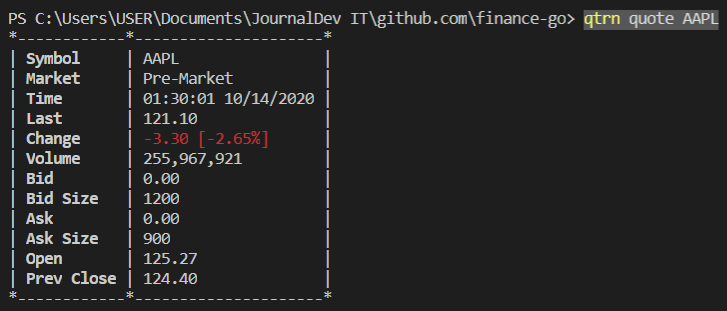
Getting Stock Option Chain in Go
We can also retrieve options, which are financial instruments that are derivatives based on the value of underlying securities such as stocks. An options contract offers the buyer the opportunity to buy or sell—depending on the type of contract they hold—the underlying asset.
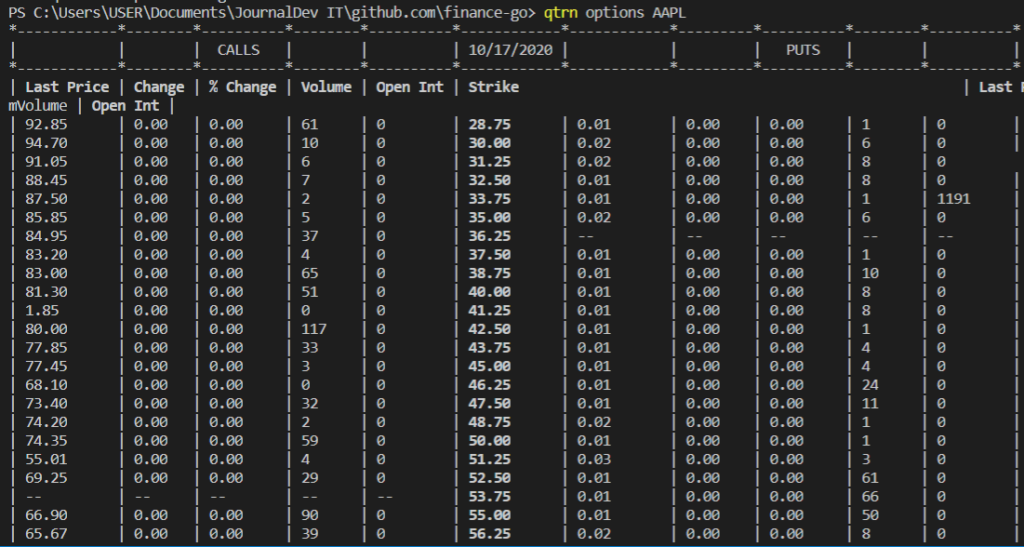
Creating Stock Price Chart in Go
Another functionality, as I mentioned, is charting stock prices for any company:
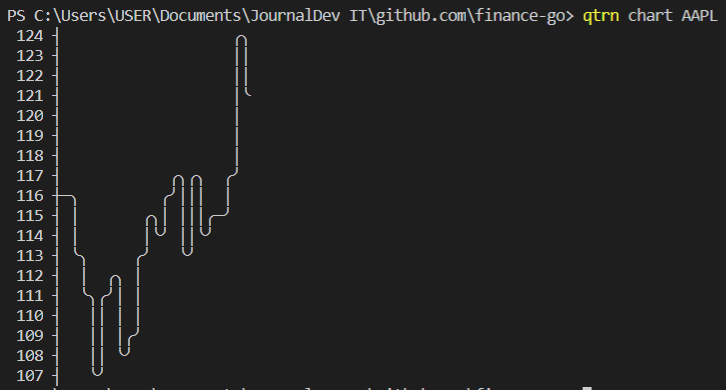
References:
- https://finance.yahoo.com/cryptocurrencies
- https://pkg.go.dev/mod/github.com/piquette/finance-go
- https://github.com/piquette/finance-go
- https://github.com/piquette/qtrn