Hello, Gophers! GORM v2.0 just released a month ago(on Aug 21, 2020), so I think it is the perfect time to look at the features of this amazing package created by a solo developer Jingzhu.
What is ORM?
So what is ORM? It stands for Object-Relational-Mapper and actually makes it easier to write code. To understand what it does, consider that we have a database that we need to do CRUD operations on. Here, CRUD stands for Create, Retrieve, Update, and Delete. Go accentuates simplicity, so it is only natural that to interact with a database, we would be using its native language, i.e, SQL, NoSQL, PostGRE, etc.
While this is fine for smaller entries and simple databases, when we start dealing with more complex database structures with lots of mapping between them, then you’ll often find yourself wasting more time on writing SQL queries than Go code. To curb this problem, we have ORMs, which you’ll find a list here.
It doesn’t however have a Go section, but we’ll cover that here anyway. These ORMs help us write fast SQL queries using the language we’re most comfortable in, and generally implementing a SQL wrapper for the language.
G-ORM – Golang ORM Package
Currently sitting at 21000 stars (!!!) on Github, gorm is a package developed mostly by Jingzhu, with a few commits from other interested individuals. It is a full-featured ORM and has several features that help us as Go devs.
Object Relationship Managers act as brokers between us developers and our underlying database technology. They allow us to essentially work with objects, much as we normally would, and then save these objects without having to craft complex SQL statements.
Gorm 2.0 has been a complete rewrite of the whole package, and has implemented several performance improvements. Let’s get started.
1. Installing G-ORM
In your terminal, simply run:
go get gorm.io/gorm
Next go into the code editor and we can simply import it in:
import (
"gorm.io/gorm"
"gorm.io/driver/sqlite"
)
Now, in the terminal, we’ll be creating a new empty file. This can be done using the touch command in Linux, or the fsutil file createnew test.db 0
command in Windows.
Mind that gorm uses SQLite in our example to access databases. So you would need to go get it. However, if that throws an error, then you need to install GCC on your computer using a ming-w installer.
2. Importing g-orm drivers
Once that’s done, create a new file main.go in your repository, and let’s import that in:
package main
import (
"gorm.io/driver/sqlite"
"gorm.io/gorm"
)
Now that that is done, we can now start. We have already created a database, so we’ll now make a struct so that we can input stuff inside in that format.
//Product ...
type Product struct {
gorm.Model
Code string
Price uint
}
3. Creating database schema
Then we can start coding our main function:
func main() {
db, err := gorm.Open(sqlite.Open("test.db"), &gorm.Config{})
if err != nil {
panic("failed to connect database")
}
// Migrate the schema
db.AutoMigrate(&Product{})
// Create
db.Create(&Product{Code: "P1", Price: 100})
This creates our schema, and we can check the documentation for how it’s implemented.
We create a product P1 for our database.
4. Golang CRUD Operations – Read, Update and Delete
We can read the product from our database using either the id or the attributes like product code:
// Read ..
var product Product
db.First(&product, 1)
db.First(&product, "code = ?", "P1")
We can update prices of products in our database quite easily:
db.Model(&product).Update("Price", 200)
Deletion of a product is also through a one-line code:
db.Delete(&product, 1)
Essentially, we are still using SQL queries but through a wrapper library which is easier to use for someone less proficient in database languages. However, if you are a pro at databases, you’d probably be able to write better-optimized code yourself. GORM has also recently been sponsored by OpenCollective, so there are better things to come.
Benchmark Performance
G-orm has also been tested against other similar ORMs on databases, and the results are worthwhile to consider:
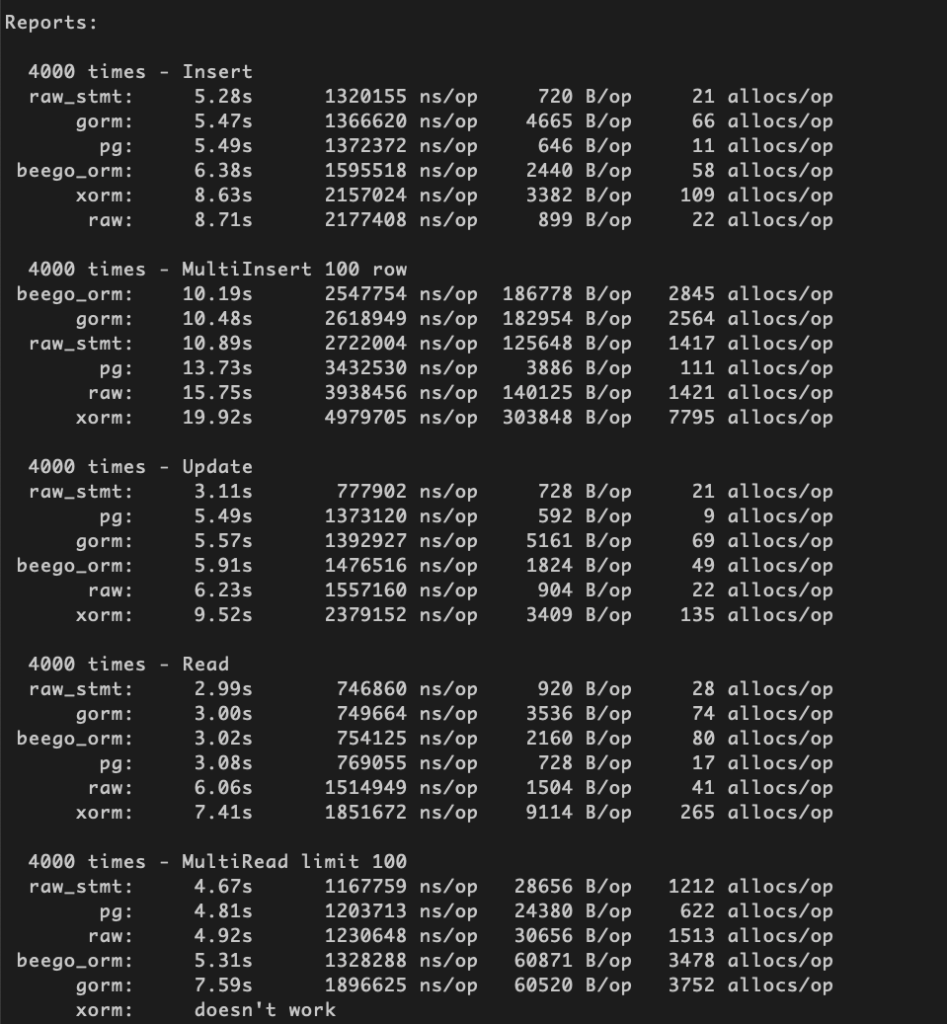