Hey, how is it going? If you like the articles, bookmark the site. If you haven’t checked out the other tutorials, you can also check those out: https://golangdocs.com/http-server-in-golang, https://golangdocs.com/golang-web-application, https://golangdocs.com/golang-graphql-gqlgen,etc.
In this article, we’ll be looking at how we can deploy any Go function to AWS Lambda super easily. We can run code for virtually any type of application or backend service – all with zero administration.
AWS Lambda and Go
Without requiring you to have or maintain servers, AWS Lambda automatically executes your Go code. Simply write your code and upload it to Lambda.
AWS Lambda dynamically scales the program in response to each input by running the code. Your code runs in parallel and independently processes each cause, correctly measuring the scale of the server workload!
With AWS Lambda, you are however charged for every 100ms your code executes and the number of times your code is triggered. You pay only for the compute time you consume. Even though it is a paid service, I am covering it because it is not only one of the most popular platforms, it is the most cost-efficient too with absolutely no server downtimes and other issues.
With AWS Lambda, you can optimize your code execution time by choosing the right memory size for your function. You can also enable Provisioned Concurrency to keep your functions initialized and hyper-ready to respond within double-digit milliseconds. Since Go is innately concurrent, they go hand in hand. In fact, it has only been recently added to the list of programming languages available (2018).
AWS Lambda interface
Well, let’s get started. This is the interface of AWS Lambda as given below. You can see that there is a create function on the top right side:

To search for this interface from AWS main homepage, just search lambda in the search bar:
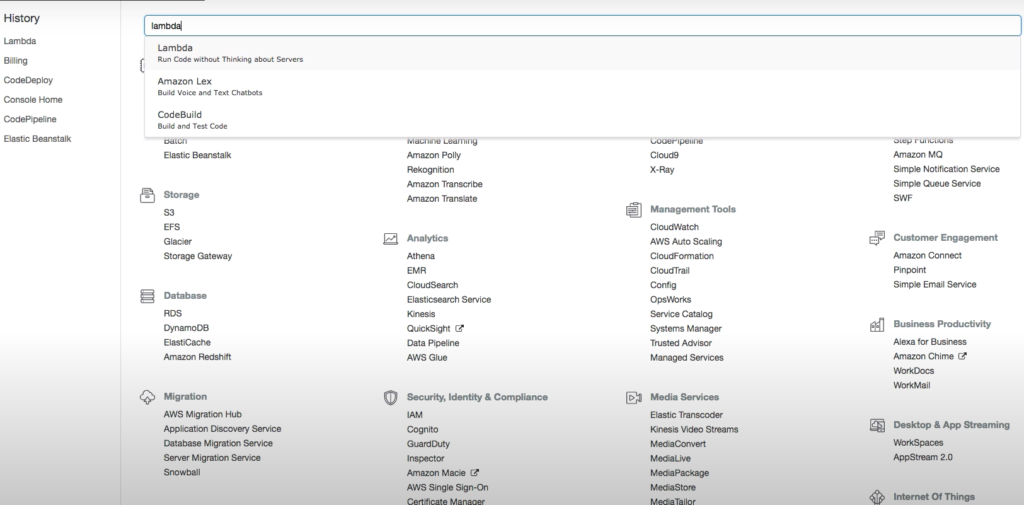
With this, we get redirected to a new screen on clicking “create function”:
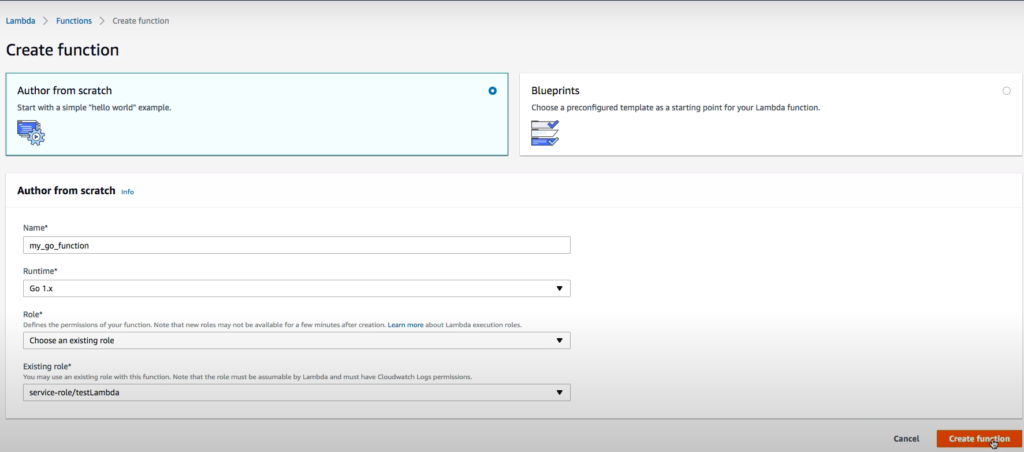
We shall give the name of our function, and then select the Runtime as Go 1.x. We can use the testLambda role. It doesn’t matter for the purpose of this tutorial.
Now, we again click on Create function, and we see a new screen:
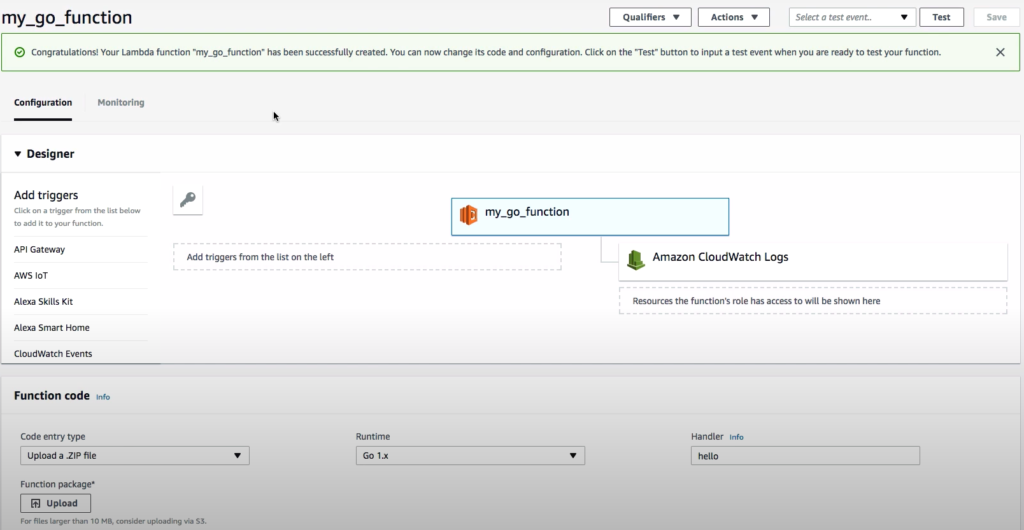
On top, it will say that our function was created successfully !
Here we can see a lot of information. Don’t add triggers, because we won’t be using any. However, as you can see, there is functionality for API, IoT, etc.
The flowchart thing simply means that all your logs from the function will go to Amazon Cloudwatch.
Below we can see that there is an option for a zip file. It doesn’t yet have an embedded code editor, so we need to put our server handler code in a .go file, and then build it into a binary and upload it here as a .zip file.
Go Code for AWS Lambda
So let’s create that now. It will be similar to the code used in our previous tutorial : Golang REST API.
package main
import (
"fmt"
"github.com/aws/aws-lambda-go/lambda"
)
We define a json struct to retrieve information of two properties – id and value:
type Request struct {
ID float64 `json:"id"`
Value string `json:"value"`
}
type Response struct {
Message string `json:"message"`
}
And then we create our handler:
func Handler(request Request) (Response, error) {
return Response{
Message: fmt.Sprintf("Process Request ID %f", request.ID),
}, nil
}
func main() {
lambda.Start(Handler)
}
Then in the terminal we write: GOOS=linux go build -o main.
Then we uploaded the created zip file, and click on test:
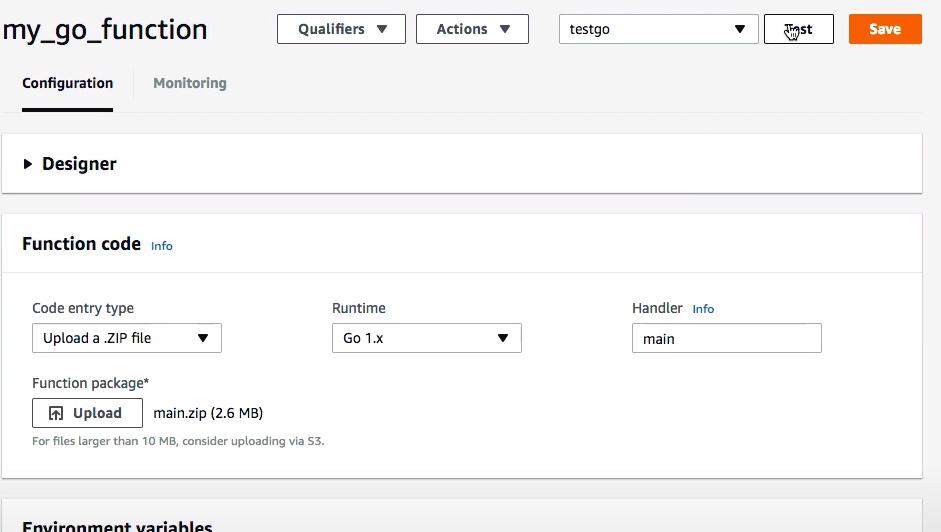
The server will test the code, and then if the run is successful you’ll get a confirmation:
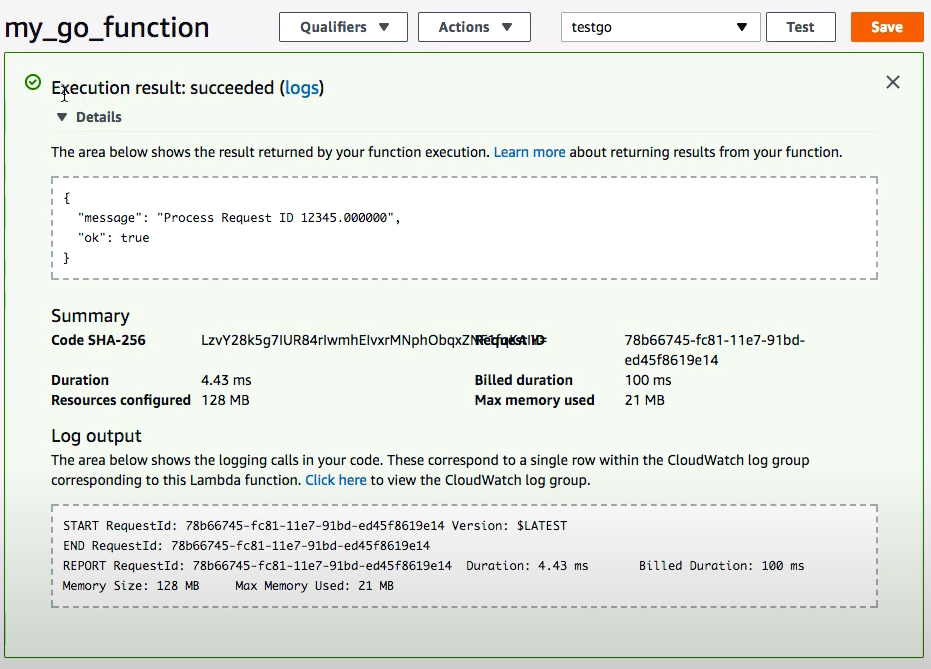