Docker is a containerization platform widely used today. Containers enable us to do things easily that was previously a hassle to do. In this post, we will containerize a simple Go application using docker.
What is Docker?
Docker is an OS-level virtualization platform that containerizes its data files and packages and isolates from other containers inside the same OS. These containers can then communicate between them in different ways such as ports.
Installing Docker
Docker can be installed pretty easily, for windows and mac the recommended is Docker Desktop. For Linux, here are instructions for ubuntu.
To check if the installation is correct open command prompt and run the following.
docker -v
This will show the output in the console.

Running this will show all the options available via docker command.
docker --help
Now, we are going to write a simple Go application.
A simple Golang HTTP Server
Here we are going to write a simple Golang http server. Create a project directory first and write the code in a file.
package main
import (
"fmt"
"io"
"net/http"
"time"
)
func MainHandler(w http.ResponseWriter, r *http.Request) {
io.WriteString(w, time.Now().Format("2006-01-02 15:04:05"))
}
func main() {
http.HandleFunc("/", MainHandler)
fmt.Println("Listening on port 5050...")
http.ListenAndServe(":5050", nil)
}
This is a simple implementation of a server that listens to port 5050 and produces string output of current local time.
If we run it, we can see:
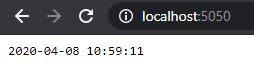
As we can see, everything works fine. Now, we will start using docker to containerise this application.
Golang Docker File
We need to create a Dockerfile for our Golang application.
Simply create a file in the project directory called Dockerfile. This name is preferred since it clearly tells what it does.
The docker file is a set of instructions that we telling docker to do when running that application. It will start from the beginning and go all the way up to running the application.
There are many commands we need to write inside that file and the first command will be the FROM command. This command creates our docker image from a pre-existing base image. That means we don’t have to waste our time setting up the base of the build. Here are some of the prebuilt images for Go. So, we will use the alpine one.
# The base go-image
FROM golang:1.14-alpine
# Create a directory for the app
RUN mkdir /app
# Copy all files from the current directory to the app directory
COPY . /app
# Set working directory
WORKDIR /app
# Run command as described:
# go build will build an executable file named server in the current directory
RUN go build -o server .
# Run the server executable
CMD [ "/app/server" ]
Building Golang Docker Application
Now, we can build the docker image of this using the following command:
docker build -t application-tag .
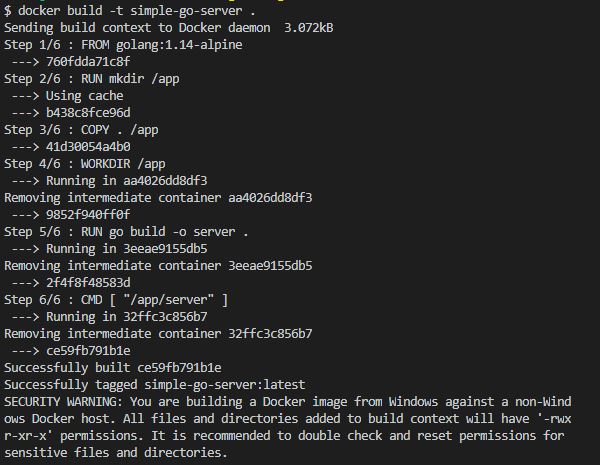
Running Golang Docker Container
Now, after we have built it, we can run it. But only running this container won’t produce results to the host OS. To see if it actually runs we need to forward the port and use the interactive mode.
The command then becomes for running:
docker run -it --rm -p 5051:5050 application-tag
The -it flag creates a interactive mode for our app so it can be seen in terminal.

Now, the application is running on post 5051 on the host OS, that has been forwarded from the port 5050 in the container.
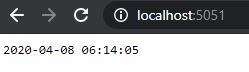
To see all running containers we can use the command inside another terminal.
docker ps

This command tells us many important info about our running containers.
Why use Docker for Golang application?
Docker is an extremely versatile containerization software that can be used with almost anything. It is also, widely supported and has a great community. Docker makes it easy for us to create a containerized app and run it in no time. This is what makes Docker such an important topic.
If you are still unsure, guess what? Docker is written in Golang. So, running a Golang application in a Docker container makes it a perfect choice.