Golang is a compiled programming language, developed and designed at Google in 2007. Its syntax is similar to that of C programming language. Writing a Go program is really easy as we will see. Today we will write our first Go program.
1. Setting up Go workspace
First of all, follow these tutorials to download and install Golang into your system.
An organized file structure is really important when programming in Golang. This is done by keeping all Go sources in one main directory. That directory is known as the workspace.
In windows that directory is %USERPROFILE%/Go. In Unix-based systems, it is set to $HOME/Go.
You can change your workspace by changing the GOPATH.
A workspace can contain multiple version-controlled repositories. These repositories can contain several packages. A package contains Go source files.
That path of the package directory is its import path.
1.1) Our workspace setup
Since our workspace directory is created already, we only need to have three subdirectories inside it. If yours have already set up, you can skip this.
Just create three subdirectories as shown below:
Go/
bin/
pkg/
src/
As you can see above there are three subdirectories, bin, pkg, and src.
1.2) Workspace hierarchy
The folder src will contain all source files and the bin folder will contain the binaries or the executable commands. The pkg directory will contain installed packages.
bin/
hello-world #command executable
other-executable #command executable
src/
github.com/userone/example-repo
.git/ #git metadata
hello-world/
hello-world.go #command source
other-executable/
main.go #command source
pkg/
Above is a sample directory structure for Go workspace.
So, let’s create our own package inside which our first source file will be.
2. Hello World Go program
So, let’s create our first Go program and execute it.
2.1) Creating our first package
Just create a directory inside src/. It will be our first package. Let’s call it go-project.
2.2) Writing our first Go code
Create a file inside that folder (in our case, go-project) and call it main.go. You can call it anything.
Now, write the code in that file. Don’t worry if you don’t understand it right now.
package main
import "fmt"
func main() {
fmt.Println("Hello World!")
}
Notice that you don’t need semicolons!
2.3) Explanation of the code
Here package main is defined above which means the program will run inside the main package.
Then in the next line, we imported a very useful package fmt using the import statement. The way to import file is:
import "packagename" // single package import
import ( // multiple package import
"packageone"
"packagetwo"
)
After importing we created a function called main. The main function is the function that runs at the start. The declaration of a function is really simple, we need to use the func keyword.
func main() {} // function declaration using func keyword
Inside the main function, we included:
fmt.Println("Hello World!")
It means we are using the Println function of fmt package to print “Hello World” in the console.
2.4) Building Go Hello World Program
Now let’s build that. In order to build it, we will use the go tool. It is a command-line tool that comes with Golang.
We need to use the command go build
inside that project directory.
go build
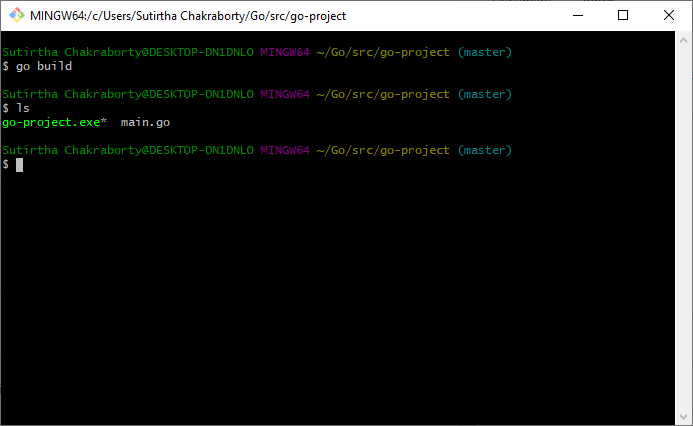
Now we will see that an executable has been created. The name of the executable will be its repository name. In our case go-project.exe.
Now, let’s execute it and see what happens. To execute that, we simply run ./filename.exe, in our case ./go-project.exe.
./go-project.exe
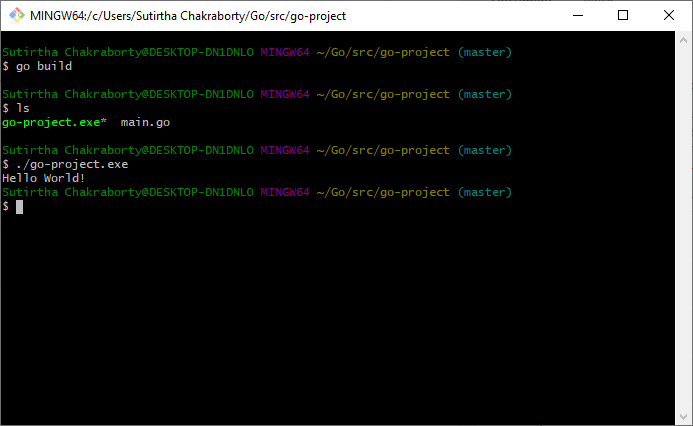
It works! Our program just printed “Hello World!” on the console. Congratulations on writing your first Go program.